728x90
1. 문제 설명
더보기
배열 array의 i번째 숫자부터 j번째 숫자까지 자르고 정렬했을 때, k번째에 있는 수를 구하려 합니다.
예를 들어 array가 [1, 5, 2, 6, 3, 7, 4], i = 2, j = 5, k = 3이라면
- array의 2번째부터 5번째까지 자르면 [5, 2, 6, 3]입니다.
- 1에서 나온 배열을 정렬하면 [2, 3, 5, 6]입니다.
- 2에서 나온 배열의 3번째 숫자는 5입니다.
배열 array, [i, j, k]를 원소로 가진 2차원 배열 commands가 매개변수로 주어질 때, commands의 모든 원소에 대해 앞서 설명한 연산을 적용했을 때 나온 결과를 배열에 담아 return 하도록 solution 함수를 작성해주세요.
2. 제한사항
더보기
- array의 길이는 1 이상 100 이하입니다.
- array의 각 원소는 1 이상 100 이하입니다.
- commands의 길이는 1 이상 50 이하입니다.
- commands의 각 원소는 길이가 3입니다.
3. 입출력예
array | commands | return |
[1, 5, 2, 6, 3, 7, 4] | [[2, 5, 3], [4, 4, 1], [1, 7, 3]] | [5, 6, 3] |
4. 풀이(과정 or 최종)
Solution 1 (내 풀이)
1. i번째에서 j번째까지 숫자를 자른것을 담기위해 ArrayList 생성
2. 정렬 후 k번째 숫자 가져와 answer에 담아주고 ArrayList 비워주기
import java.util.*;
class Solution {
public int[] solution(int[] array, int[][] commands) {
int[] answer = new int[commands.length];
List<Integer> list = new ArrayList<>();
for(int i=0; i < commands.length; i++){
int start =commands[i][0]-1;
int end = commands[i][1];
for(int j=start; j<end; j++){
list.add(array[j]);
}
list.sort(Comparator.naturalOrder());
answer[i] = list.get(commands[i][2]-1);
list.clear();
}
return answer;
}
}
Solution 2 ( Arrays.copyOfRange )
import java.util.*;
class Solution {
public int[] solution(int[] array, int[][] commands) {
int[] answer = new int[commands.length];
for(int i=0; i<commands.length; i++){
int[] temp = Arrays.copyOfRange(array, commands[i][0]-1, commands[i][1]);
Arrays.sort(temp);
answer[i] = temp[commands[i][2]-1];
}
return answer;
}
}
Solution 3 ( 퀵정렬 구현)
class Solution {
public int[] solution(int[] array, int[][] commands) {
int n = 0;
int[] ret = new int[commands.length];
while(n < commands.length){
int m = commands[n][1] - commands[n][0] + 1;
if(m == 1){
ret[n] = array[commands[n++][0]-1];
continue;
}
int[] a = new int[m];
int j = 0;
for(int i = commands[n][0]-1; i < commands[n][1]; i++)
a[j++] = array[i];
sort(a, 0, m-1);
ret[n] = a[commands[n++][2]-1];
}
return ret;
}
void sort(int[] a, int left, int right){
int pl = left;
int pr = right;
int x = a[(pl+pr)/2];
do{
while(a[pl] < x) pl++;
while(a[pr] > x) pr--;
if(pl <= pr){
int temp = a[pl];
a[pl] = a[pr];
a[pr] = temp;
pl++;
pr--;
}
}while(pl <= pr);
if(left < pr) sort(a, left, pr);
if(right > pl) sort(a, pl, right);
}
}
5. 결과
Solution 1
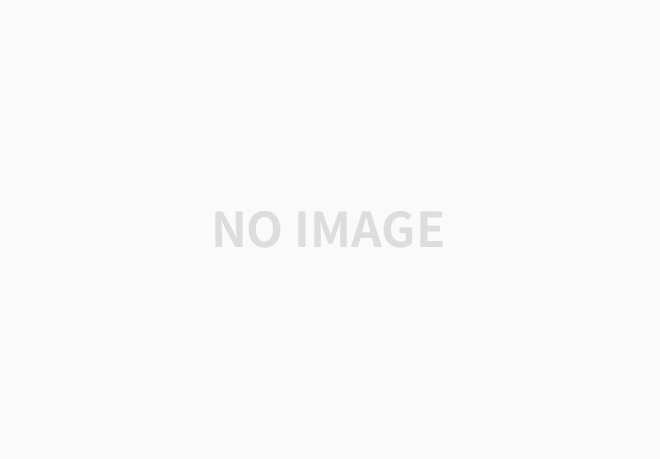
Solution 2
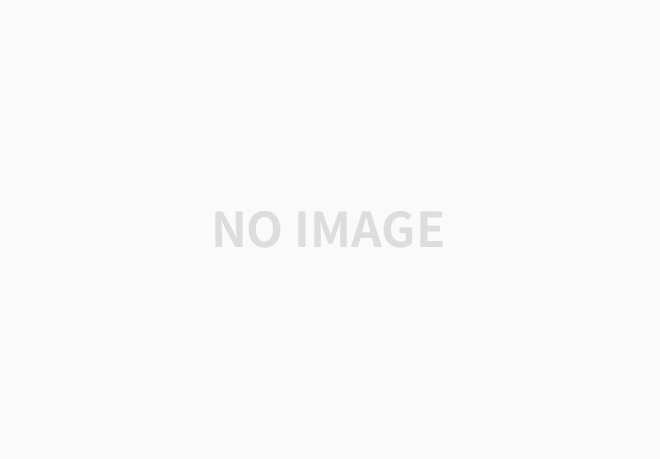
Solution 3
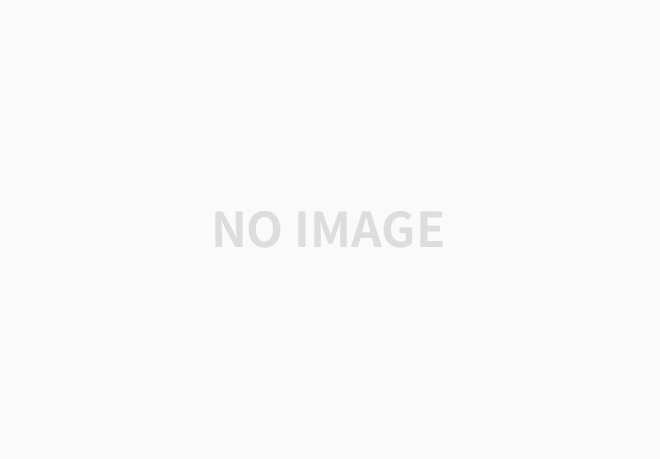
6. 마치며
쉬운거 후딱 풀어보려고 했는데 생각보다 배울게 너무 많은 문제였다.
라이브러리/메소드를 쓰지 않은 코드가 제일 빠르고 제일 공부가 잘 되는 코드가 아닐까 싶다. (대단👏)
배운건 따로 포스팅 하였다.
https://firstblog912.tistory.com/150
[JAVA] 배열복사 메서드 ( Arrays.copyOf(Range))
자바에서 메서드를 사용하지 않고 배열을 복사하는 방법은 for문이나 while문 같은 반복문을 활용하여 직접 값을 하나씩 넣어주는 것이다. 그 보다 간단하게 메서드를 사용해서 배열을 복사하는
firstblog912.tistory.com
반응형
'공부 > 알고리즘' 카테고리의 다른 글
[프로그래머스] 완주하지 못한 선수 (0) | 2022.06.15 |
---|---|
[프로그래머스] 조이스틱 (0) | 2022.06.12 |
[프로그래머스] 더 맵게 (0) | 2022.06.10 |
[프로그래머스] 네트워크 (0) | 2022.06.09 |
[프로그래머스] 예산 (0) | 2022.06.06 |
댓글